Overview
The StoreAPI is a GraphQL API. This will be your main way of communicating with our backend. Here you can fetch things like categories, products and pages. You can learn more about GraphQL in the official documentation
What is GraphQL
GraphQL is a query language and a server-side runtime (typically served over HTTP) - GraphQL
Graph
The biggest and coolest thing with GraphQL are the graphs. Graphs are structures that look like the way we construct models mentally.
Graphs are powerful tools for modeling many real-world phenomena because they resemble our natural mental models and verbal descriptions of the underlying process. With GraphQL, you model your business domain as a graph by defining a schema; within your schema, you define different types of nodes and how they connect/relate to one another. On the client, this creates a pattern similar to Object-Oriented Programming: types that reference other types. On the server, since GraphQL only defines the interface, you have the freedom to use it with any backend (new or legacy!) - GraphQL.
Queries and Mutations
While perhaps not strictly correct, you could view queries and mutations as endpoints in a REST API. Queries are used for getting data from the backend, while mutations are used for posting data to the backend. Clicking one of the queries/mutations will reveal information about what variables it accepts and what type it returns.
Types
A type can be Scalar (Int, Float, String, Boolean, etc.) or Object, such as Product
and Category
. An object graph type has fields, these fields would also be scalar or object types. As an example, lets say a Category
object graph type has the following fields: id:String, name:String and products:[Product]
. "id" and "name" would be of scalar type String
, while "product" would be a list of object type Product
. Product
would then in turn have it's own set of fields. This nesting of object graph types is what makes up the Graph in GraphQL.
Interfaces
We would encourage you to read the official documentation. But in a nutshell, just know that when a field is an interface type, it can be one of potentially multiple object types (implementations). These objects will have some common fields. One example would be Customer
, Customer
is an interface and can be either a PrivateCustomer
or BusinessCustomer
object type. The fields will be different depending on the object type. PrivateCustomer
has a "pid" field while BusinessCustomer
has an "organizationId" field. All other fields are the same. You can observe this in the schema explorer: Customer(query) -> data:Customer(type)
Highlight difference between REST and GraphQL
Think in graphs, not endpoints - GraphQL
Over- and under fetching
As in REST queries you fetch full contracts, whether you want the entire contract or not, you will get it all. Or maybe you only want one field from a contract, but you must fetch the entire anyway. This is called over fetching, but let's say that an endpoint does not provide enough of the required data. Then the client would have to make another endpoint request to fetch the remaining required data, this is the under fetching-part of REST. You will probably end up over- and under fetching data from the REST API, through no fault of its own. In GraphQL you don't work with endpoints that way, you simply ask for the contract's fields you are interested in.
Here is a example in GraphQL where one only want to get the products name, the campaigns it's in and price:
query MyProductQuery {
product(articleNumber: "1337") {
name
campaigns{name}
price{incVat}
}
}
And here is the output:
{
"data": {
"product": {
"name": "Leet Lamp",
"campaigns": ["Summer Campaign"],
"price": {
"incVat": 13.37
}
}
}
}
Whilst in REST you would have to fetch the entire product object in order to just get these three fields.
Multiple endpoints vs one endpoint
To highlight another difference between REST API and GraphQL, we will use a example below:
REST API:
GraphQL:
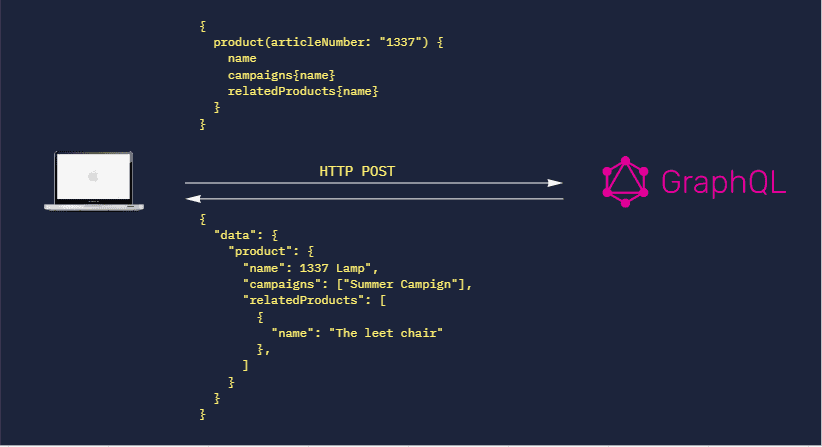
As you clearly can see, you will have to use a HTTP Post to GraphQL to fetch all fields you want. Unlike REST API where you will have to use three difference endpoints to get the fields you need.
Official documentation
GraphQL - https://www.graphql.com